ذخیره داده های لاروال در Elastic و RabbitMQ
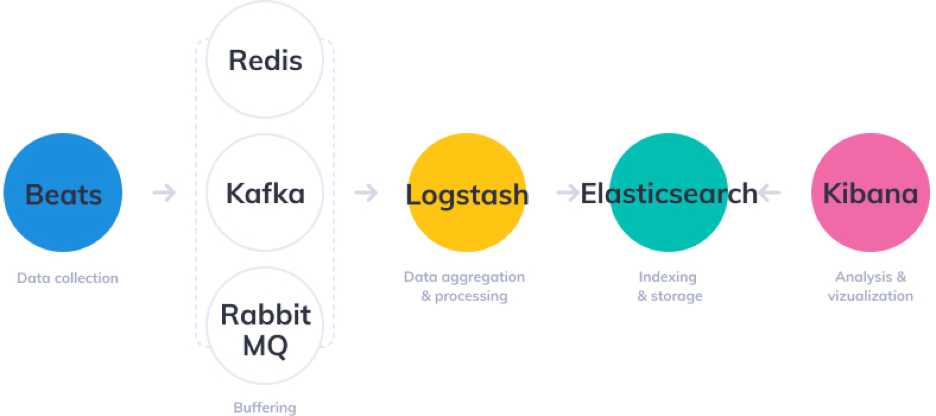
در این پست بطور خلاصه ذخیره اطلاعات از لاروال به RabbitMQ و از آن به Elasticsearch با استفاده از logstash رو بطور خیلی خلاصه بررسی میکنیم.
در اینجا از RabbitMQ به منظور حفظ داده ها در زمان لود بالای elastic و یا غیرفعال بودن سرویس به منظور جلوگیری از دست رفتن داده استفاده میکنیم.
docker run -d --hostname rabbit --name rabbit -e RABBITMQ_DEFAULT_USER=admin -e RABBITMQ_DEFAULT_PASS=admin -p 15672:15672 -p 5672:5672 docker.arvancloud.ir/rabbitmq:3-management
ایجاد Exchange و Queue در RabbitMQ
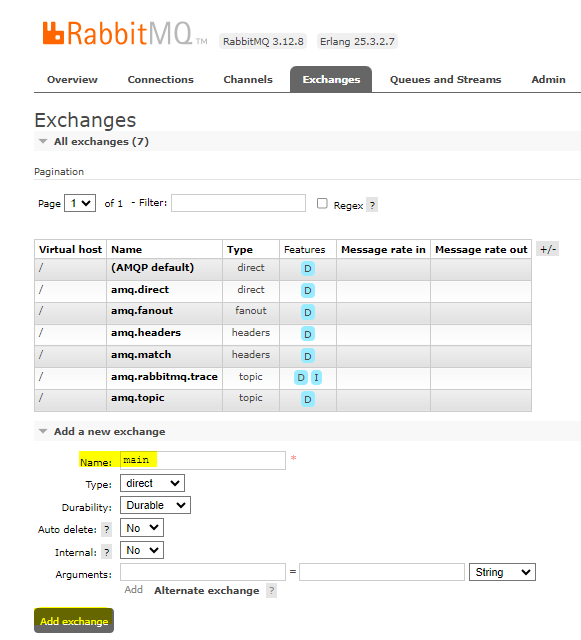
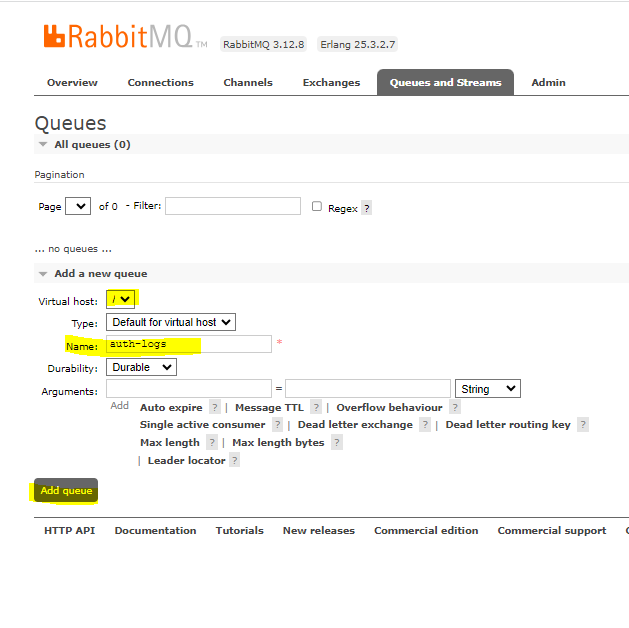
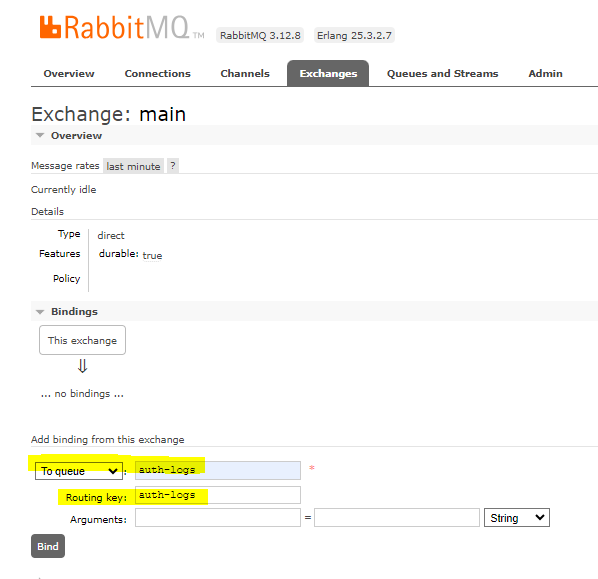
اضافه کردن کدها
composer require php-amqplib/php-amqplib
.env
RABBITMQ_HOST=127.0.0.1
RABBITMQ_PORT=5672
RABBITMQ_VHOST=/
RABBITMQ_LOGIN=admin
RABBITMQ_PASSWORD=admin
nano app/RabbitMQService.php
<?php
namespace App;
use PhpAmqpLib\Connection\AMQPStreamConnection;
use PhpAmqpLib\Message\AMQPMessage;
class RabbitMQService {
protected $connection;
protected $channel;
public function __construct()
{
$this->connection = new AMQPStreamConnection(
env('RABBITMQ_HOST'),
env('RABBITMQ_PORT'),
env('RABBITMQ_LOGIN'),
env('RABBITMQ_PASSWORD'),
env('RABBITMQ_VHOST')
);
}
public function publish($msg,$values)
{
$this->channel = $this->connection->channel();
$this->channel->exchange_declare($values["exchange"], 'direct', false, true, false);
$this->channel->queue_declare($values["queue"], false, true, false, false);
$this->channel->queue_bind($values["queue"], $values["exchange"], $values["key"]);
$msg = new AMQPMessage($msg);
$this->channel->basic_publish($msg,$values["exchange"], $values["key"]);
}
public function __destruct() {
$this->channel->close();
$this->connection->close();
}
}
استفاده از تابع در کنترلرهای مدنظر
use App\RabbitMQService;
---
$message = $request->input('message');
$values = [
'exchange' => 'main',
'queue' => 'auth-logs',
'key' => 'auth-logs'
];
$rabbitMQService = new RabbitMQService();
$rabbitMQService->publish($message,$values);
return response('Message published to RabbitMQ');
ایجاد logstash pipeline برای ارسال لاگها به Elastic
nano /opt/logstash/rabbit.conf
input {
rabbitmq {
id => "input_rabbit"
host => "127.0.0.1"
port => 5672
user => "admin"
password => "admin"
exchange => "main"
exchange_type => "direct"
queue => "auth-logs"
durable => true
key => "auth-logs"
prefetch_count => 2000
threads => 1
tags => input_rabbit
}
}
output {
if "input_rabbit" in [tags]{
elasticsearch {
user => admin
password => admin
ssl => true
ssl_certificate_verification => false
index => auth-logs
}
}
}
docker run -d --hostname logstash --name logstash -it --add-host=elastics:172.17.0.1 -v /opt/logstash/rabbit.conf:/usr/share/logstash/pipeline/rabbit.conf docker.arvancloud.ir/logstash:8.10.2
مطلبی دیگر از این انتشارات
همه چیز در مورد نحوه استفاده از Repository pattern در فریم ورک Laravel
مطلبی دیگر از این انتشارات
فیلامنت، ورود با نام کاربری به جای ایمیل
مطلبی دیگر از این انتشارات
فیلامنت، اضافه کردن صفحه ویرایش پروفایل