برنامه نویس.42ساله. از مدرک MCSD دات نت سال 2002 شروع کردم البته بعد از لیسانس و تمام عمرم رو در مدیریت با ابزار های شیرپوینت و MSPS و CRM و غیره گذراندم.https://zil.ink/sabert
شبیه سازی ارسال وب هوک تلگرام و بله برای برنامه نویسی روبات
از چت جی پی تی پرسیدم:
بهم بگو چطوری بهتره وب هوک هایی که از سمت سرور تلگرام و بله ارسال میشه رو در محیط برنامه نویسی development شبیه سازی کنم. که قابل تکرار باشه و با همون ساختار بشه TDD تست هم نوشت و انقدر وابسته به وب هوک ها در سرور نباشیم و بتونیم انواع اقسام قالب های جیسون که ارسال میشه از سرور تلگرام به سمت سرور ما رو هندل کنیم و کاور کنیم و پوشش بدیم.
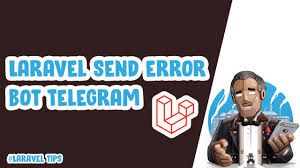
To simulate all webhooks and their JSON payloads for your Laravel application, you can follow these detailed steps. This involves setting up routes, creating controllers, and writing a script to send simulated webhook requests.
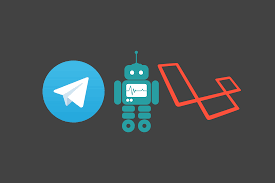
### Step 1: Set Up Webhook Routes
First, define routes in your Laravel application to handle webhook requests. For example:
```php
// routes/web.php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\WebhookController;
Route::post('/webhook/{provider}', [WebhookController::class, 'handleWebhook']);
```
### Step 2: Create a Webhook Controller
Create a controller to handle incoming webhook requests and log them for debugging purposes:
```php
// app/Http/Controllers/WebhookController.php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Log;
class WebhookController extends Controller
{
public function handleWebhook(Request $request, $provider)
{
// Log the incoming request for debugging
Log::info('Webhook received from ' . $provider, $request->all());
// Process the webhook payload as per your logic
// ...
return response()->json(['status' => 'success']);
}
}
```
### Step 3: Create a Command to Simulate Webhooks
You can create an Artisan command to simulate sending webhooks. This is particularly useful for testing purposes.
#### Step 3.1: Generate a Command
Run the following Artisan command to generate a new command:
```sh
php artisan make:command SimulateWebhooks
```
#### Step 3.2: Implement the Command
Edit the generated command file to include logic for sending webhook requests:
```php
// app/Console/Commands/SimulateWebhooks.php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\Http;
class SimulateWebhooks extends Command
{
protected $signature = 'simulate:webhooks';
protected $description = 'Simulate sending webhooks to the application';
public function handle()
{
$webhookUrl = 'http://your-app.test/webhook/sample-provider'; // Change this to your local or testing URL
$webhookPayloads = [
[
'event' => 'user.created',
'data' => [
'id' => 1,
'name' => 'John Doe',
'email' => 'john@example.com',
],
],
[
'event' => 'user.updated',
'data' => [
'id' => 1,
'name' => 'John Doe',
'email' => 'john.doe@example.com',
],
],
// Add more payloads as needed
];
foreach ($webhookPayloads as $payload) {
$response = Http::post($webhookUrl, $payload);
if ($response->successful()) {
$this->info('Webhook sent successfully: ' . json_encode($payload));
} else {
$this->error('Failed to send webhook: ' . json_encode($payload));
}
}
$this->info('All webhooks have been simulated.');
}
}
```
### Step 4: Run the Simulation Command
Finally, run the command to simulate sending webhooks:
```sh
php artisan simulate:webhooks
```
### Additional Considerations
- **Testing Environment**: Ensure that your webhook URL points to the correct environment (e.g., local development server).
- **Logging**: Check your `storage/logs/laravel.log` file to see the logged webhook requests and responses.
- **Payloads**: Customize the `webhookPayloads` array to include all the different webhook events and payloads you need to test.
This setup allows you to simulate webhook requests and see how your application handles them, helping you ensure that your webhook processing logic works correctly under different scenarios.
مطلبی دیگر از این انتشارات
نوشتن پلاگین اکتنشن برای گوگل کروم. چرایی و چگونگی
مطلبی دیگر از این انتشارات
لاراول Nova ادمین پنل دانلود نصب استفاده
مطلبی دیگر از این انتشارات
بورد اسکرام کاغذی. مزایا.